- Learnings from the homework
- Displaying, reading and parsing information from the web
While reviewing this week’s homework we came across the use of double arrays (I could not find documentation for these types of arrays on the processing website). These arrays store two dimensions of data in a matrix that can be visualized as a spreadsheet or grid (check out the fig. a below). These arrays are the basis for image and video processing since to the computer a display is nothing more than a two-dimensional array.
- Syntax for declaring double arrays: “arrayType [][] arrayName;”
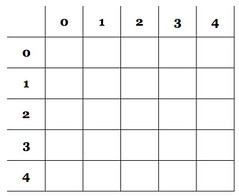
Another interesting type of array is the ArrayList. These are a special type of array that is dynamically allocated. What this means from a practical perspective is that you can add and remove elements while the program is running. An array lists is a more efficient solution than appending normal arrays. However, if you know how many elements you will need before run time, it is most efficient to use a standard array.
This power comes at a price, to use data from these arrays you need to create temporary local variables that are often not necessary when using regular arrays. An array list also has a different set of function than a normal array (even the ones that perform the same functions have different names,).
- Syntax for declaring an array list: “ArrayList arrayName;”
- Syntax for creating the array list: “arrayName = new ArrayList();”
- Syntax for adding element to array list “arrayName.add(new Class());” - the “new Class()” in this example can be replaced by a primitive date type such as int, char, etc.
- Syntax for extracting data from an array list: “Class tempVarName = (classOfArrayElement) arrayName.get(ArrayElementNumber);"
Displaying text information
During the second half of this week’s class we learned how to handle text - both how to display text and how to read text data from sources such as the Internet. I can’t wait to begin using these capabilities since data visualization is one of the areas that I hope to explore during my time at ITP. Let’s start with the basics and work our way up.
Definition of characters and strings:
- Characters (chars) is a data type for typographic characters, such as ‘a’, ‘b’, ‘c’ , and you get my drift. Chars are represented as ‘single’ quotes.
- Strings (String) is a data type that contains an array of chars. Unlike traditional arrays you don’t need to define the size of your string when you create it. Strings are represented by “double” quotes.
- Declare a variable of type PFont
- Define the variable usingloadFont() or createFont(). Do this during the sketch set-up because it requires a good amount of time.
- Use textFont() function to set the font type and size that you want to use for a single use of the text().
- Display text using the text() function. Method has several arguments: string, x and y coordinates, and width and length (optional).
On the other hand, createFont() enables to load a font at the moment the application is running. It also supports resizing of fonts without pixilation. However, if the person running the application does not have the selected font then the system defaults to an available font, which may look horrible with your design.
Capturing information from the Internet
For the most part, being able to take data from a website and using that data to impact a sketch takes a lot of detective work. The reason being you need to look at the source code from a website to understand how it is structured so that you can determine if there is a standard syntax that can enable your sketch to consistently find the information you want to capture. Here is an overview of the entire process
1. In practice this first step involves looking for identifiers within HTML/XML code that precede and follow the golden nugget of information you seek. It is always easier to use XML feeds than HTML code (and firefox is better to investigate XML than Safari). If using XML code you should leverage processing’s XML library. Once you have found the source on the internet from which you want to import data go to the next step.
2. Load the page into processing as a string using the loadStrings(URL) function. This method loads the document into an array of strings, each line is put into a separate element in the array. This means that when we are declaring a variable to import a text document we need to set that variable as an array – “string [] lines = loadString(sampleURL);”
3. Next use the join() function to put the contents of this array into a single string. The syntax for the join() function is “Join(string array to be joined, element to add between array elements)”. For example join(htmlPageArray, “ “) would join all the lines from a string array with a space between each element in the array.
4. Use string parsing functions described below to locate the data we want within an XML or HTML document/code. All of these methods tare part of the String class).
Parcing information from the Internet
First off you need to find the location of the data you are looking for by using the unique identifiers that you discovered during step one described above. Then you will use this information to extract data from the string. Remember that you can use a loop to look for multiple similar elements on a page. Here is the step-by-step:
- The function stringVar.indexOf(subStringSearch) returns the location within the stringVar where substring starts. If the subStringSearch is not found then it returns a -1 value. In order to find the start location of a sub string we need to add the length of the subStringSearch variable to value returned by this function.
- Once you have the location of the data use the stringVar.subString(start position inclusive, end position exclusive) to get the sub string that exists between the start and end point. It is important to always keep in mind how the start and end points of this function are handled differently.
No comments:
Post a Comment